This post shows a simple example of creating a simple stateless EJB that has two business methods. One generates a random number, between zero and one, and the other method takes a single parameter that will generate a number between zero and that number.
- Under the NetBeans IDE, create a new Java EE Enterprise Application project, give it a name, set the location and click Next.
- Set the server as JBoss Application Server, Java EE version 5 and create an EJB module with the name RandomNumber-ejb and click Finish.
A new project appears on the Projects list as shown below.
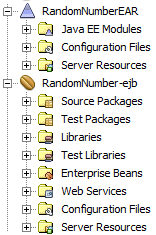
New EJB Project
Right click on the RandomNumber-ejb project, and
- Select new Session Bean
- Give it a name of RandomNumber and a package name
- Set it to Stateless
- Select the Local and Remote checkboxes
- Click Finish
A new EJB appears in the list called RandomNumber. Open this in the source editor.
Create two new methods,
- findNum that takes no arguments and returns a double
- findNumInRange that takes a double as an argument and returns a random double between 0 and that number.
To create a method and ensures it gets promoted to the Remote interface
- Right click in the source editor
- Select Insert Code…
- Select Add Business Method…
- A dialog box appears to enter the method name, parameters and return types.
Once implemented, right click on the EAR project and select Clean and Build… and then deploy it by selecting the Deploy option. JBoss will deploy the EJB and make it available as shown in the logs:
11:24:47,403 INFO [SessionSpecContainer] Starting jboss.j2ee:ear=RandomNumberEAR.ear,jar=RandomNumber-ejb.jar,name=RandomNumber,service=EJB3
11:24:47,403 INFO [EJBContainer] STARTED EJB: com.riz.randomnumber.beans.RandomNumber ejbName: RandomNumber
11:24:47,872 INFO [JndiSessionRegistrarBase] Binding the following Entries in Global JNDI:
RandomNumber - EJB3.x Default Remote Business Interface
RandomNumberEAR/RandomNumber/remote-com.riz.randomnumber.beans.RandomNumberRemote - EJB3.x Remote Business Interface
Now that the EJB is deployed, you can create a client to test the EJB under JBoss, but we will make web services out of these via JAX-WS and then generate clients to call the web services.
To create a web service from an EJB, right click the bean under the EJB module project,
- Select New > Web Service…
- Name the web service and give it a package name
- Select the option Create Web Service from Existing Session Bean
- Browse for the EJB you created, select it and click Finish.
The web service should be created and can be viewed in the project hierarchy:
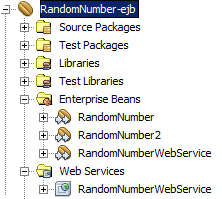
Web service view
Deploy the EAR project onto JBoss server, the logs should show it deployed.
11:24:48,434 INFO [SessionSpecContainer] Starting jboss.j2ee:ear=RandomNumberEAR.ear,jar=RandomNumber-ejb.jar,name=RandomNumberWebService,service=EJB3
11:24:48,434 INFO [EJBContainer] STARTED EJB: com.riz.randomnumber.ws.RandomNumberWebService ejbName: RandomNumberWebService
11:24:48,863 INFO [JndiSessionRegistrarBase] Binding the following Entries in Global JNDI:
11:24:48,906 INFO [DefaultEndpointRegistry] register: jboss.ws:context=RandomNumberEAR-RandomNumber-ejb,endpoint=RandomNumberWebService
11:24:49,111 INFO [WSDLFilePublisher] WSDL published to: file:/C:/jboss-5.1.0.GA/server/default/data/wsdl/RandomNumberEAR.ear/RandomNumber-ejb.jar/RandomNumberWebServiceService5116477380875990466.wsdl
11:24:49,184 INFO [TomcatDeployment] deploy, ctxPath=/RandomNumberEAR-RandomNumber-ejb
11:24:49,200 WARNING [config] Unable to process deployment descriptor for context '/RandomNumberEAR-RandomNumber-ejb'
11:24:49,363 INFO [config] Initializing Mojarra (1.2_12-b01-FCS) for context '/RandomNumberEAR-RandomNumber-ejb'
11:24:49,386 INFO [TomcatDeployment] deploy, ctxPath=/RandomNumber-war
11:24:49,459 INFO [Http11Protocol] Starting Coyote HTTP/1.1 on http-127.0.0.1-8889
11:24:49,479 INFO [AjpProtocol] Starting Coyote AJP/1.3 on ajp-127.0.0.1-8818
The web services that are deployed on the server can be browsed by pointing to the jbossws context root, http://localhost:8080/jbossws (your port number should be changed if different to default 8080).
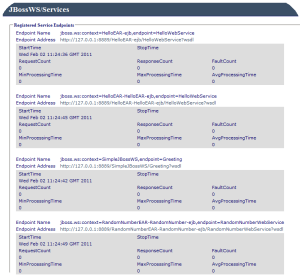
JBoss deployed web services
Notice that the WSDLs can be browsed for each web service that is deployed. The RandomNumber web service has now been deployed and is present on the list of services. A web service client now needs to be implemented to make use of this deployed service.
- Make a note of the URL of the WSDL of the RandomNumber web service.
- Create a new Web Project (if the client is web based) or Java Application (is standard J2SE application)
- Start DOS (under Windows) or shell (under Linux)
- Navigate to the project source folder cd <project location>\src\java
- Now JBoss needs to generate client-side code based on the WSDL deployed so that clients can invoke the web services. This is done via the JBoss wsconsume tool.
- Invoke the wsconsume tool by issuing this command (for web project, Windows)
c:\jboss-5.1.0.GA\bin\wsconsume.bat -k -s . -o ..\..\build\web\WEB-INF\classes -t 2.1 http://127.0.0.1:8889/RandomNumberEAR-RandomNumber-ejb/RandomNumberWebService?wsdl
Output is shown when this command is executed:
generating code...
com\riz\randomnumber\ws\FindNum.java
com\riz\randomnumber\ws\FindNumInRange.java
com\riz\randomnumber\ws\FindNumInRangeResponse.java
com\riz\randomnumber\ws\FindNumResponse.java
com\riz\randomnumber\ws\ObjectFactory.java
com\riz\randomnumber\ws\RandomNumberWebService.java
com\riz\randomnumber\ws\RandomNumberWebServiceService.java
com\riz\randomnumber\ws\package-info.java
compiling code...
<other output>
Go back to the NetBeans editor and the source packages should now have an additional package that contains the generated web service client code.
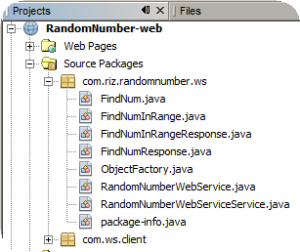
NetBeans source package view
Create a new servlet and enter this snippet of code to call the web service that has been deployed on JBoss:
protected void processRequest(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
RandomNumberWebServiceService service = new RandomNumberWebServiceService();
RandomNumberWebService port = service.getRandomNumberWebServicePort();
double maxNumber = 5.0;
out.println("<html>");
out.println("<head>");
out.println("<title>Servlet RandomNumberServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>");
out.println("no arg call: " + port.findNum());
out.println("one arg call: " + port.findNumInRange(maxNumber));
out.println("</h1>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
The code snippets in red are the web service calls.
Deploy this application on JBoss and run the servlet, the following should be displayed in a web browser:
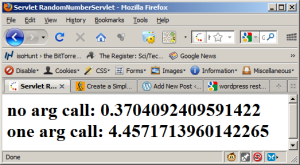
Servlet output
That’s all there is to it to create simple web or J2SE clients to call deployed web services on JBoss.